Getting the data from a solar panel inverter
Purpose and setup
To use solar energy direct while it is produced, some sockets of our power users are switched "on" or "off" by a programmable microcontroller. In our case we use an Arduino Uno and a Solax X1 inverter.
The relay of the boiler socket is hard wired to the Arduino and for some other users we use remote controlled (433MHz) sockets.
To know when to switch the users, internal data of the inverter is used.
My main interest is the delivered power in Watts for the switching and the temperature to provide cooling with an external fan.
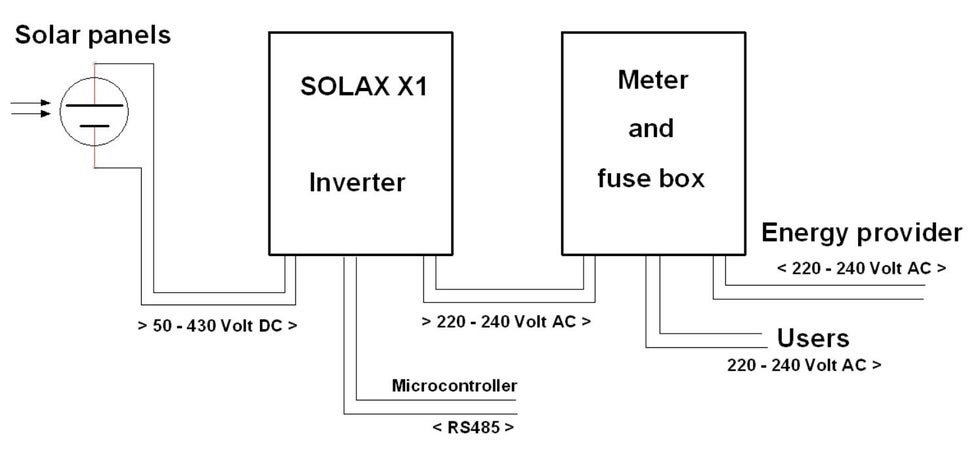
The inverter
To transform the 50–430 V DC from the solar panels to the 220-240 V AC of the power grid, a Solax X1 inverter is used.
During the process heat is generated .
The internal data of the inverter can be reached via a RS485 communication port with a RJ 45 Jack.
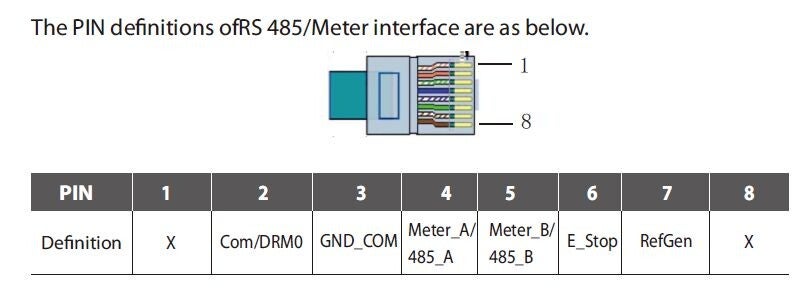
The RS485 data transfer is a differential system with two data lines A and B. (Pin 4 and 5 on the RJ45 plug) They are not grounded to be more resistant to interference. If line A is 5 volts, line B is 0 volts and vice versa. In our system, a MAX 485 module, connected to our Arduino microprocessor, converts the data stream into TTL with 5 volts relative to ground.
Communication protocol
The inverter uses a MODBUS protocol and has the be asked for its data. The master (our Arduino microprocessor) sends a request for the data and the slave (The inverter) replies. The data packets contain a sender- and a destination- address.
The Solax protocol can be downloaded from the web and I used this version:
"SolaxPower_Single_Phase_External_Communication_Protocol_X1_V1.8.pdf"
In this protocol AP stands for access point - the master initiating the data transfer.
Request the serial number from the Iinverter
To communicate I define an array of bytes which is send through the max module
SoftwareSerial RS485Serial(SSerialRX, SSerialTX);
byte InByte = 0;
byte byteInput[25];
byte RequestSerialNumber[]=
{0xAA,0x55,0x01,0x00, 0x00,0x00, 0x10, 0x00, 0x00, 0x01,0x10};
The request is send as follows:
RS485Serial.write(RequestSerialNumber,sizeof(RequestSerialNumber));
And the response from the Inverter is catched in the byteInput[25]:
int index = 0;
while(RS485Serial.available())
{
InByte = (byte)RS485Serial.read();
byteInput[index] = InByte;
index++;
}
Assigning the address to the inverter
The received serial number is used to assign an address to inverter:
byte InByte = 0;
byte ConformationInput[12]; // the conformation send by the inverter is 12 bytes long
byte AddressInput[] = {0xAA,0x55,0x00,0x00, 0x00,0x00, 0x10, 0x01, 0x0F,
0x31,0x32,0x33,0x34,0x35,0x36,0x37,0x37,0x36,0x35,0x34,0x33,0x32,0x31, 0x0A,0x04,0x01};
In the main controlling program I use this array in an "AssignAddress()" function :
void AssignAddress()
{
digitalWrite(REPin, RS485Transmit);
digitalWrite(DEPin, RS485Transmit);
RS485Serial.write(AddressInput,sizeof(AddressInput));
delay(100);
// * * * SWITCH TO RECEIVE MODE * * *
digitalWrite(REPin, RS485Receive);
digitalWrite(DEPin, RS485Receive);
int index = 0;
while(RS485Serial.available())
{
InByte = (byte)RS485Serial.read();
ConformationInput[index] = InByte;
index++;
}
}
The address assignment has to be done each time the inverter has been switched off (which happens at night). So if I want to use inverter data, I put the address asignment in the setup function of the program and start the Arduino in the morning.
Requesting the data
The RequestData[] array and DataInput are defined as:
byte RequestData[]={0xAA,0x55, 0x00,0x00, 0x00,0x0A , 0x11, 0x02, 0x00, 0x01,0x1C};
byte DataInput[63];
In the loop() of the program I use a function to make my data requests and receive the data packet:
void GetSolaxData()
{
// * * * SEND A DATA REQUEST TO THE INVERTER * * *
digitalWrite(REPin, RS485Transmit);
digitalWrite(DEPin, RS485Transmit);
RS485Serial.write(RequestData,sizeof(RequestData));
// * * * SWITCH TO RECEIVE MODE AND UPDATE THE DATAPACKET * * *
digitalWrite(REPin, RS485Receive);
digitalWrite(DEPin, RS485Receive);
int index = 0;
while(RS485Serial.available())
{
InByte = (byte)RS485Serial.read();
DataInput[index] = InByte;
index++;
}
// update the data values of interest
power_Solax = DataInput[28] + 256 * DataInput[27];
temp_Solax = DataInput[10];
voltage_Grid = (DataInput[24] + 256 * DataInput[23]) / 10.0;
}
The data values are each 2 bytes HEX values. Some data fit in one byte like the temperature in DataInput[10].
The power is given in Watts and need both bytes as it mostly exceeds 256 watts.
For example:
DataInput[27] = 0x06 = Decimal 6
DataInput[28] = 0xBF = Decimal 191
The power_Solax = 6 x 256 + 191 = 1747 Watts.
This is plenty to run our electric boiler of 1500 Watts so I can switch the socket of the boiler "on".
The complete program listings are written in a way that they provide info on your serial monitor while running.
They can easily be adopted to your own situation and needs;
_250226_A_Solax_RequestSerialNumber
_250226_B_Solax_AssignAddress Sends address to Inverter and gets conformation
_250226_C_Solax_RequestData Shows the full data packet on your monitor
Important note:
_250226_C_Solax_RequestData and the assigning program _250226_B_Solax_AssignAddress should run on the same day as the Solax inverter doesn't store the assignment.
In my controlling program the assignment is done during the setup() of the program. Be aware that you have to start the program each day as the inverter switches off during the night an will loose the address assignment. You can do this manually or put the Arduino on a timer.
Jeroen Droogh bootprojecten@gmail.com